Integration of Single Hyperbox-based Models with Sklearn Pipeline and Hyperopt
This example shows how to integrate the GFMM classifier into the Pipeline class implemented by scikit-learn
Note that this example is illustrated by using the original online learning algorithm for GFMM model. However, it can be used for any GFMM models using other learning algorithms
[1]:
import warnings
warnings.filterwarnings('ignore')
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import MinMaxScaler
from sklearn.model_selection import train_test_split
from hbbrain.numerical_data.incremental_learner.onln_gfmm import OnlineGFMM
Load Iris dataset and prepare training and testing sets
[2]:
from sklearn.datasets import load_iris
[3]:
df = load_iris()
X = df.data
y = df.target
[4]:
X_train, X_test, y_train, y_test = train_test_split(X, y, train_size=0.8, random_state=0)
Create a pipeline of pre-processing method (i.e., normalization of data in the range of [0, 1]) and a GFMM classifier.
Note: The GFMM classifier using an original online learning algorithm requires the input data in the range of [0, 1].
[5]:
theta = 0.1
theta_min = 0.1
onln_gfmm_clf = OnlineGFMM(theta=theta, theta_min=theta_min)
[6]:
pipe = Pipeline([('scaler', MinMaxScaler()), ('onln_gfmm', onln_gfmm_clf)])
Training
[7]:
pipe.fit(X_train, y_train)
[7]:
Pipeline(steps=[('scaler', MinMaxScaler()),
('onln_gfmm',
OnlineGFMM(C=array([2, 1, 0, 2, 2, 1, 0, 1, 1, 1, 2, 0, 0, 1, 2, 2, 2, 2, 1, 2, 2, 2,
1, 2, 1, 1, 2, 0, 1, 0, 0, 1, 0, 1, 2, 2, 0, 2, 0, 2, 1, 1, 0, 2,
2, 0, 0, 2, 2, 1, 1, 0, 1, 0, 2, 1]),
V=array([[0.58333333, 0.41666667, 0.68965517, 0.70833333],
[0.30555556, 0.41666667, 0.5862069 , 0.58333333],
[0.19444444, 0.625 , 0.05172414, 0.04166667],
[0.44444444, 0.416666...
[0.86111111, 0.33333333, 0.86206897, 0.75 ],
[0.38888889, 0.25 , 0.44827586, 0.375 ],
[0.66666667, 0.41666667, 0.67241379, 0.66666667],
[0.19444444, 0.41666667, 0.0862069 , 0.04166667],
[0.44444444, 0.5 , 0.63793103, 0.70833333],
[0.33333333, 0.625 , 0.03448276, 0.04166667],
[0.55555556, 0.375 , 0.77586207, 0.70833333],
[0.41666667, 0.29166667, 0.51724138, 0.375 ]]),
theta=0.1, theta_min=0.1))])
Testing
[8]:
acc = pipe.score(X_test, y_test)
print(f'Testing accuracy = {acc * 100: .2f}%')
>>> The testing sample 26 with the coordinate [ 0.08333333 0.66666667 -0.01724138 0.04166667] is outside the range [0, 1]. Membership value = 0.916667. The prediction is more likely incorrect.
Testing accuracy = 96.67%
The example below shows how to use the HyperOpt library in combination with PipleLine and Cross-validation to find the best model
[9]:
from hyperopt import fmin, hp, tpe, Trials, space_eval, STATUS_OK
from hyperopt.pyll import scope as ho_scope
from hyperopt.pyll.stochastic import sample as ho_sample
Define search space
[10]:
hp_space_gfmm = {
'theta': hp.uniform('theta', 0, 1),
'gamma': hp.uniform('gamma', 0, 10)
}
# Draw random sample to see if hyperspace is correctly defined
ho_sample(hp_space_gfmm)
[10]:
{'gamma': 4.542173063848446, 'theta': 0.8064001273117817}
Defining model
[11]:
def init_model(hps):
"""
Constructs estimator
Parameters:
----------------
hps : sample point from search space
Returns:
----------------
model : sklearn.Pipeline.pipeline with hyperparameters set up as per hps
"""
# Assembing pipeline
model = Pipeline([
('scale', MinMaxScaler()),
('clf', OnlineGFMM(**hps))
])
return model
[12]:
from sklearn.model_selection import cross_val_score, StratifiedKFold
from sklearn.metrics import accuracy_score
Now we have to define function to minimize. We’ll stick with cross-validation score on train set. Our function should take a sample from search space and return negative mean Acc score. As noted above, it is very important to return negative score here, since otherwise we’ll seek for hyperparameters that minimize Acc
[13]:
def f_to_min1(hps, X, y, ncv=5):
"""
Target function for optimization
Parameters:
----------------
hps : sample point from search space
X : feature matrix
y : target array
ncv : number of folds for cross-validation
Returns:
----------------
: target function value (negative mean cross-val Acc score)
"""
model = init_model(hps)
cv_res = cross_val_score(model, X, y, cv=StratifiedKFold(ncv, shuffle=True, random_state=0),
scoring='accuracy', n_jobs=1)
return -cv_res.mean()
Running optimization
[14]:
from functools import partial
import numpy as np
All right, let’s run optimization for 100 rounds using TPE algorithm, meaning that we use TPE to suggest next sample values based on previous function evaluations. We’ll use Trials class objects to keep track of optimization history. Note: We’re binding X and y arguments of target function to X_train and y_train respectively, using functools.partial, since target function of fmin may accept only a search space point.
[15]:
trials_clf = Trials()
best_clf = fmin(partial(f_to_min1, X=X_train, y=y_train),
hp_space_gfmm, algo=tpe.suggest, max_evals=100,
trials=trials_clf, rstate = np.random.default_rng(0))
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.888627. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.945905. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.842222. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.800702. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.701052. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.837797. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.921216. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.899729. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.709743. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.564614. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.414332. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.799199. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.414332. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.260208. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.814764. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.910028. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.885491. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.668525. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.502788. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.227886. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.663078. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.227886. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.024698. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.633662. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.822064. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.773537. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.344448. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.016672. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.417466. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.717055. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.174743. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.971811. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.987699. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.957717. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.946590. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.946590. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.917323. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.971654. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.917323. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.895566. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.843349. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.586375. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.793187. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.379562. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.310625. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.216289. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.595076. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.823306. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.595076. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.232775. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.232775. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.536979. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.797955. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.536979. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.415131. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.122697. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.225142. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.734335. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.225142. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.527092. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.770302. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.707657. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.153744. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.207652. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.728338. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.207652. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.484989. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.823425. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.484989. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.024189. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.024189. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.673487. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.836743. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.510230. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.381343. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.381343. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.738973. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.886097. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.738973. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.505423. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.505423. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.505573. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.759850. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.694354. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.115235. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.585234. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.857794. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.585234. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.476084. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.214127. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.650744. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.825372. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.476116. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.338252. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.338252. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.679227. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.839613. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.518840. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.392219. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.392219. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.666495. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.833248. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.499743. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.368097. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.368097. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.847477. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.923738. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.847477. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.711009. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.711009. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.869514. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.934757. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.869514. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.752763. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.752763. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.977404. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.990140. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.977404. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.957186. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.957186. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.792677. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.896339. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.792677. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.607178. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.607178. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.876281. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.946013. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.876281. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.765584. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.765584. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.849137. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.934169. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.849137. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.748562. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.714154. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.912143. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.961663. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.912143. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.833535. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.833535. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.810589. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.905295. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.715884. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.684315. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.641116. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.939373. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.969686. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.909059. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.885127. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.885127. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.937304. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.968652. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.905956. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.881207. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.881207. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.719306. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.877516. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.719306. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.532177. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.468160. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.398965. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.737730. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.398965. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.389053. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.733405. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.389053. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.448095. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.759169. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.448095. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.457615. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.763323. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.186423. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.551708. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.782258. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.364920. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.197794. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.659884. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.834801. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.789746. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.391371. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.087057. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.627892. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.837625. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.627892. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.379819. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.294953. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.649130. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.824565. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.473694. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.335193. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.335193. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.277219. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.684605. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.708327. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.899998. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.708327. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.631571. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.447357. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.683243. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.846147. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.551261. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.433172. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.149758. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.760300. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.917817. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.760300. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.697221. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.545832. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.690040. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.845020. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.535061. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.412708. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.412708. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.805245. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.902623. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.707868. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.630991. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.630991. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.620962. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.870044. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.620962. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.521215. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.281822. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.906224. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.959079. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.906224. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.822319. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.822319. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.864965. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.941076. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.864965. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.744145. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.744145. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.251775. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.625888. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.172844. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.586422. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.532322. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.772842. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.566335. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.163103. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.415407. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.744905. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.123111. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.025679. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.431079. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.751744. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.146619. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.051799. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.496359. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.780230. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.496359. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.045733. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.045733. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.518529. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.766143. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.702363. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.138421. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.999140. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.999625. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.999140. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.998913. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.998370. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.559096. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.807605. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.559096. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.164603. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.164603. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.379206. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.729108. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.068810. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.377855. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.728518. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.066782. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.224555. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.612278. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.959355. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.980258. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.942419. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.927266. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.890899. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.516105. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.834093. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.516105. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.388765. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.083147. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.356339. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.719130. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.356339. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.753394. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.876697. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.753394. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.532747. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.532747. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.957302. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.978651. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.935953. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.919098. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.919098. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.973522. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.986761. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.960283. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.949831. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.949831. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.997910. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.999088. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.997910. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.996040. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.996040. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.821118. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.910559. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.731677. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.661065. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.661065. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.886690. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.943345. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.886690. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.785307. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.785307. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.194951. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.597475. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.459018. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.763935. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.188527. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.933546. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.971002. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.933546. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.874086. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.874086. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.726992. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.863496. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.726992. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.482722. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.482722. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.604519. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.827427. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.604519. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.250668. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.250668. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.716278. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.858139. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.574417. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.462421. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.462421. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.795196. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.897598. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.692794. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.611951. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.611951. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.656288. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.828144. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.484432. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.348757. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.348757. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.825042. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.923655. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.825042. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.668500. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.668500. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.771271. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.921579. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.771271. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.711079. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.566619. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.696148. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.867410. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.696148. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.493580. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.424281. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.566989. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.811050. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.566989. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.453039. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.179558. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.658673. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.829336. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.488009. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.353275. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.353275. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.854032. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.927016. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.854032. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.723428. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.723428. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.775829. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.902180. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.775829. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.575256. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.575256. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.898866. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.955869. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.898866. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.808378. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.808378. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.687186. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.863499. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.687186. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.407299. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.407299. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.636231. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.841264. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.636231. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.310753. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.310753. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.607021. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.865264. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.607021. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.503605. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.255408. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.814544. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.909921. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.885354. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.668131. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.502196. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.683919. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.841960. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.525879. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.401110. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.401110. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.284114. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.687613. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.284114. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.809766. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.934777. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.809766. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.759704. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.639556. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.888344. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.951277. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.832516. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.813907. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.788442. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.394295. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.735692. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.394295. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.000000. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.840977. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.930608. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.840977. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.734961. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.698693. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.478630. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.739315. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.478630. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.012141. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.012141. The prediction is more likely incorrect.
>>> The testing sample 19 with the coordinate [1.05882353 0.75 0.9137931 0.79166667] is outside the range [0, 1]. Membership value = 0.540064. The prediction is more likely incorrect.
>>> The testing sample 11 with the coordinate [-0.02857143 0.41666667 -0.01818182 0. ] is outside the range [0, 1]. Membership value = 0.770032. The prediction is more likely incorrect.
>>> The testing sample 21 with the coordinate [0.94285714 0.25 1.03636364 0.91666667] is outside the range [0, 1]. Membership value = 0.540064. The prediction is more likely incorrect.
>>> The testing sample 6 with the coordinate [ 0.19444444 -0.10526316 0.4137931 0.375 ] is outside the range [0, 1]. Membership value = 0.128542. The prediction is more likely incorrect.
>>> The testing sample 12 with the coordinate [0.38888889 1.15789474 0.06896552 0.125 ] is outside the range [0, 1]. Membership value = 0.128542. The prediction is more likely incorrect.
100%|█████████████████████████████████████████████| 100/100 [00:16<00:00, 6.08trial/s, best loss: -0.9666666666666666]
[16]:
# Building and fitting classifier with best parameters
clf = init_model(space_eval(hp_space_gfmm, best_clf)).fit(X_train, y_train)
# Calculating performance on validation set
clf_val_score = accuracy_score(y_test, clf.predict(X_test))
print('Cross-val score: {0:.5f}; testing score: {1:.5f}'.\
format(-trials_clf.best_trial['result']['loss'], clf_val_score))
print('Best parameters:')
print(space_eval(hp_space_gfmm, best_clf))
>>> The testing sample 26 with the coordinate [ 0.08333333 0.66666667 -0.01724138 0.04166667] is outside the range [0, 1]. Membership value = 0.478630. The prediction is more likely incorrect.
Cross-val score: 0.96667; testing score: 0.96667
Best parameters:
{'gamma': 6.256443392850102, 'theta': 0.09273471494941352}
[17]:
def f_wrap_space_eval(hp_space, trial):
"""
Utility function for more consise optimization history extraction
Parameters:
----------------
hp_space : hyperspace from which points are sampled
trial : hyperopt.Trials object
Returns:
----------------
: dict(
k: v
), where k - label of hyperparameter, v - value of hyperparameter in trial
"""
return space_eval(hp_space, {k: v[0] for (k, v) in trial['misc']['vals'].items() if len(v) > 0})
def f_unpack_dict(dct):
"""
Unpacks all sub-dictionaries in given dictionary recursively. There should be no duplicated keys
across all nested subdictionaries, or some instances will be lost without warning
Parameters:
----------------
dct : dictionary to unpack
Returns:
----------------
: unpacked dictionary
"""
res = {}
for (k, v) in dct.items():
if isinstance(v, dict):
res = {**res, **f_unpack_dict(v)}
else:
res[k] = v
return res
[18]:
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
from matplotlib.animation import FuncAnimation
import seaborn as sns
[19]:
fig0 = plt.figure(figsize=(12, 10))
gs = gridspec.GridSpec(nrows=3, ncols=1, hspace=0.5,wspace=0.3)
# ================================
# Plotting optimization history
ax = fig0.add_subplot(gs[0, 0])
ax.plot(range(1, len(trials_clf) + 1), [-x['result']['loss'] for x in trials_clf],
color='red', marker='.', linewidth=0)
ax.set_xlabel('Iteration', fontsize=12)
ax.set_ylabel('Accuracy', fontsize=12)
ax.set_title('Optimization history', fontsize=14)
ax.grid(True)
# ================================
# Plotting sampled points
samples = [f_unpack_dict(f_wrap_space_eval(hp_space_gfmm, x)) for x in trials_clf.trials]
ax = fig0.add_subplot(gs[1, 0])
sns.histplot(x=[x['gamma'] for x in samples], bins=10, ax=ax)
ax.set_xlabel('$gamma$', fontsize=10)
ax.set_ylabel('Counts', fontsize=10)
ax.grid(True)
# ----------------
ax = fig0.add_subplot(gs[2, 0])
sns.histplot(x=[x['theta'] for x in samples], bins=10, ax=ax)
ax.set_xlabel('$theta$', fontsize=12)
ax.set_ylabel('Counts', fontsize=12)
ax.grid(True)
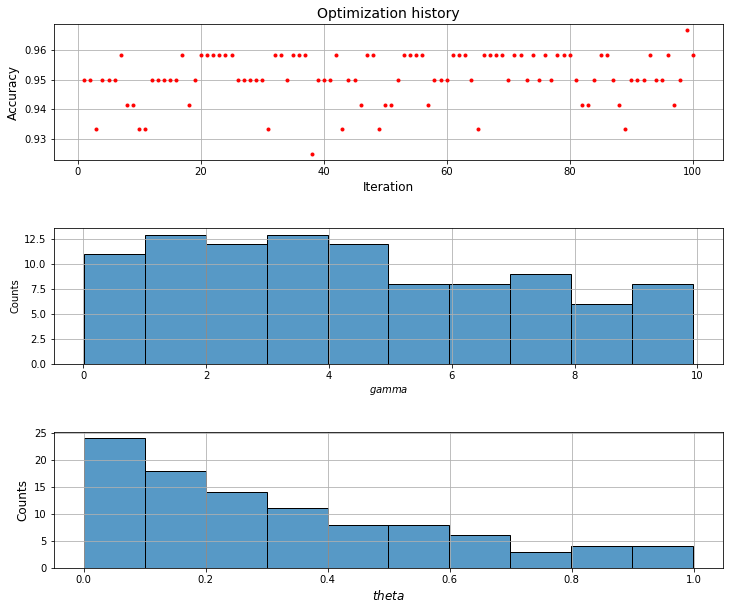